Arrays and For-Loops
[Practical 3 of 11 - Part 1]
Introducing Arrays:
An array is a container that has a single label. A visual representation of a simple array can be seen in Figure 1. The container has many elements and each element can contain a different value (in this case V1 to V5). We can access the different elements using the index for each one.
The index for elements in an array always starts at 0. To access the value at element 5, the index 4 would be used. To access the first element the index 0 would be used. When an array is created the number of elements it contains, it's length, is fixed and cannot be changed. The array label is the name that is given to the array when it is created.
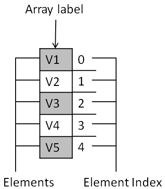
Declaring Arrays:
When an array is created there are three stages.
- Declaration, the array is declared and it's type specified.
- Construction, the length of the array is provided.
- Initialisation, when the array size has been specified values can be put into the elements.
- Declare the array on one line, construct it on another and then initialise the elements.
- Declare and construct the array on one line and then initialise the elements.
- Declare, construct and initialise the array all on one line.
Declare, Construct and Initialise Separately:
We are going to tidy up our car factory and use an array to keep all of our Car
objects
together.
In the CarFactory.java file we used in the last practical, delete all of the code between the line that prints the Started and Finished messages to the screen.
Your file should look like the one in Figure 2 when you have finished.
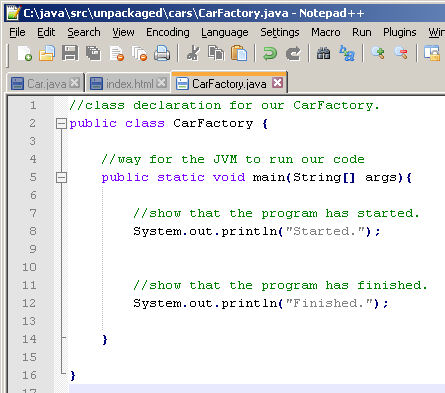
Insert the code highlighted in Figure 3 into your CarFactory.java file. Compile the code and run the program as we did in the last practical
Oh dear something has gone wrong, see Figure 4! When the code tried to run it caused an error, but it compiled fine. This is called a run-time error, it happened when we tried to run the code after it had compiled successfully. So what happened?
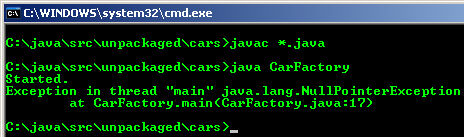
On the first line we declared an array called cars
of type Car
which is fine.
Arrays can hold primitive data types or objects and Car
is the class on which all
Car
objects are built. So that is OK.
On the second line we construct the array cars
to be of length 3
and we
are setting the elements to be new Car
objects. Wrong this is a common
mistake when people new to Java start learning about arrays. What this line is doing is creating a
new array object to hold 3 Car
type objects.
That's right an array is an object too. A very special one because we don't declare it like we would
a normal object. We never mention the class Array
anywhere in the declaration. It is the
[]
that tell the JVM that this is an Array object. The object type or primitive that we
use in the declaration and construction lines tell the JVM what we are going to store in the array.
This means that we haven't actually put anything into the array because the new
keyword
referred to the array itself. That is where the problem lies.
The error message java.lang.NullPointerException
means that there was no value,
null
, at a point where a value was expected. The next line of the message at
CarFactory.main(CarFactory.java:17)
is telling us that the error happened on line
17 of the CarFactory.java file.
If we look at line
17 in the CarFactory.java file in Figure 3 we can see that this
is where we are writing the contents of our first element to screen. However, there is nothing in
the element so we get an error. All we need to do is initialise the cars
array with
some Car
objects to fix the problem and that is what we will do next.
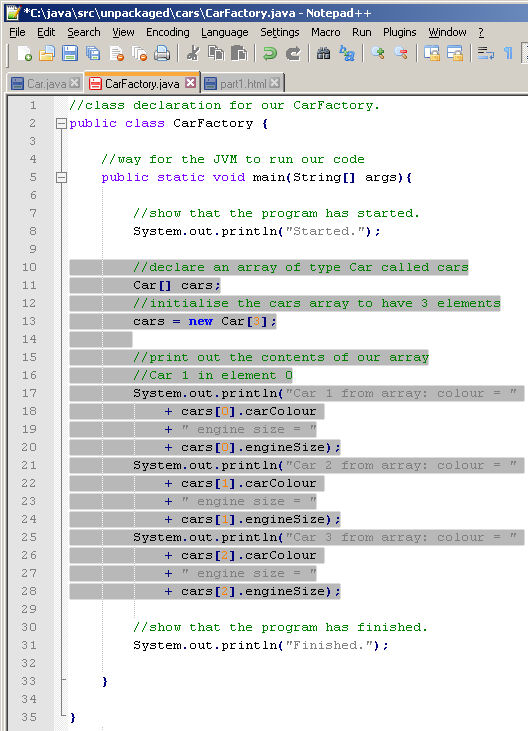
Insert the code highlighted in Figure 5 after the array construction but before we start accessing
our Car
objects to initialise the three elements. What do you think the output of the
program will be now? Save, compile and run the program as before and see what you get.
You should get three car outputs printed to the screen all containing the default engine size and colour as you can see in Figure 6.
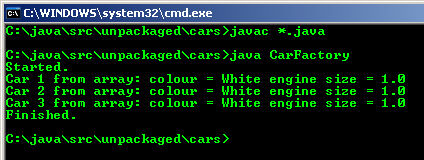
That is our first array successfully declared, constructed and initialised! These are all
new Car()
objects but we can put existing objects into an array as long as they are
the correct object type or primitive.
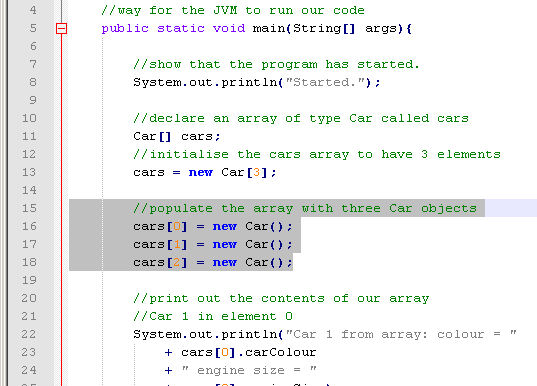
For example, lets create our car1
object from the previous practical and put that into
element 1 instead of the new Car()
. Insert and adjust the highlighted lines
of code shown in Figure 7.
Save, compile and run your program. The car colour and engine size for the first
Car
element in our array is equal to that of the object we created on
line 16 and setup on lines 17 and 18.
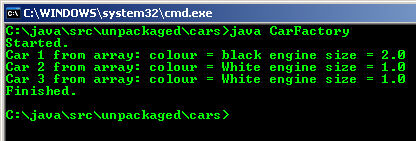
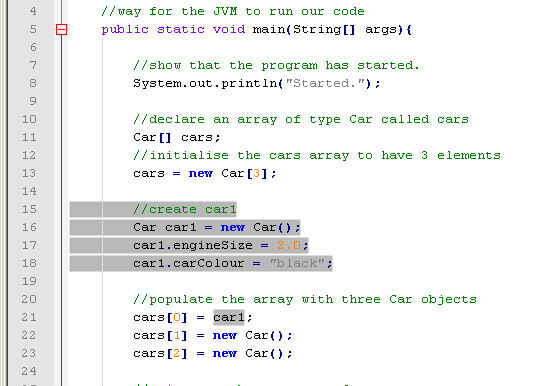
We can also access our Car
objects and set them up once they are in the array.
Insert the highlighted lines of code shown in Figure 9 after the array has been populated.
Save, compile and run your program. The car colour and engine size for the second
Car
element in our array has changed to reflect the setup we specified in the additional
lines of code added as shown in Figure 10.
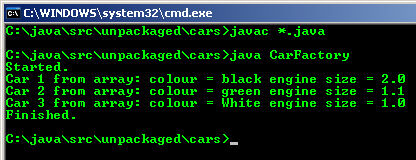
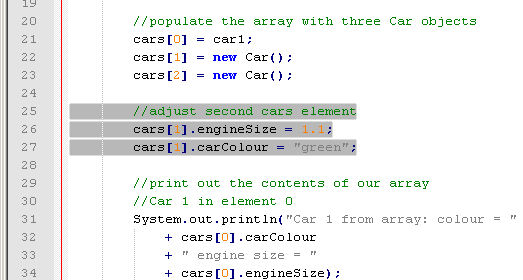
Declaration Details:
The type we use on declaration must match the type on construction or else your code will not
compile. If we declare our array as Car[] cars;
and then construct as cars = new
String[3];
you would get an error shown in Figure 11. This is called a
compile-time error, we didn't get to run-time because the compiler
found an issue with our code and reported it to us.
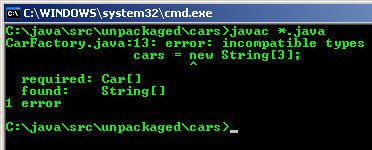
However, it doesn't matter to the compiler where in the declaration line the []
are.
These two lines are equivalent in the declaration Car[] cars;
and
Car cars[];
. It makes no difference where you put the []
to the compiler
and both these options will run, try using this line Car cars[];
on line 11
of your program. When you save, compile and run there should be no difference.
You can functionally put a white space preceding the []
but that is regarded as bad
practice as it makes the code harder to read. The accepted convention is to use the form
Car[] cars;
to declare an array.
Declare and Construct On Same Line:
The second way to create an array is to declare and construct on the same line. To do this we need to know the type and size of the array we want to create when we declare it.
Figure 12 shows the CarFactory.java file adjusted to show this type of declaration. The lines we used to declare and construct the array in Figure 3 (lines 11 and 13) have been adjusted and entered on one line.
It is important to note that the declaration and construction stages are still happening, it is the way we specify for these two stages to happen that has changed.
There is not a great deal of difference between these two array declaration forms, and both are quite common.
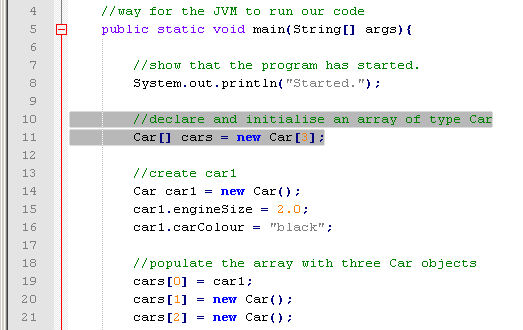
Declare, Construct and Initialise On Same Line:
The third way to create an array is to declare, construct and initialise the array on the same line. To do this we need to know the type and contents of the array we want to create when we declare it.
Figure 13 shows the CarFactory.java file adjusted to show this type of declaration.
The line we used to declare and construct the array in Figure 12 (line 11) has been
removed, as have the lines used to initialise the array (lines 19 to 21).
Replacing all of these is one single line, Car[] cars = {car1, new Car(), new Car()};
,
between the declaration of car1
and the setup of the first element in the array shown
highlighted in Figure 13.
Again, it is important to note that the declaration, construction and initialisation stages are still happening. This declaration method just provides a convenient way to specify all three stages in one line if we know the contents of the array when we declare it.
This form of declaration is very different and less common than the previous two.
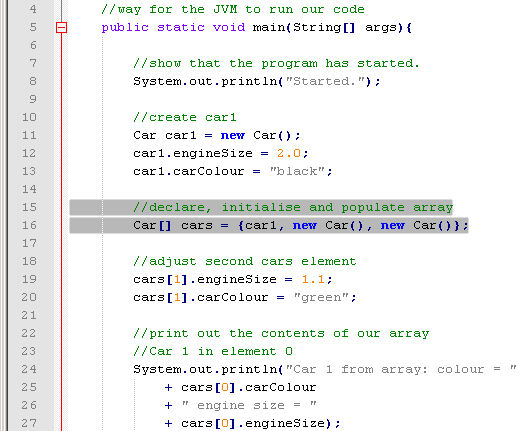