Arrays and For-Loops
[Practical 3 of 11 - Part 2]
The Length Attribute:
To find the length of an array if it is not known we can use an attribute called length
.
We use this in a similar way to the engineSize
and carColour
attributes in
our Car
class.
Add the lines highlighted in Figure 14 to the end of the CarFactory.java file just before the line which prints out that the program has finished. Save, compile and run the program. You should get an output like the one shown in Figure 15.
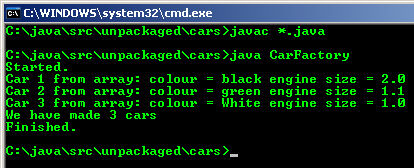
The length attribute is accessed using the .
operator as we have been doing to change
read the attributes of the Car
class.
An important point to note is that the length
attribute of an array cannot be changed
after the array has been constructed. Attempting to do so will result in a
compile-time error as shown in Figure 16.
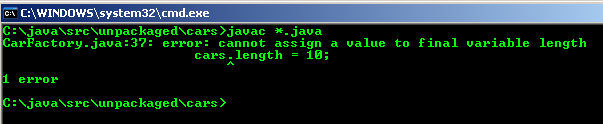
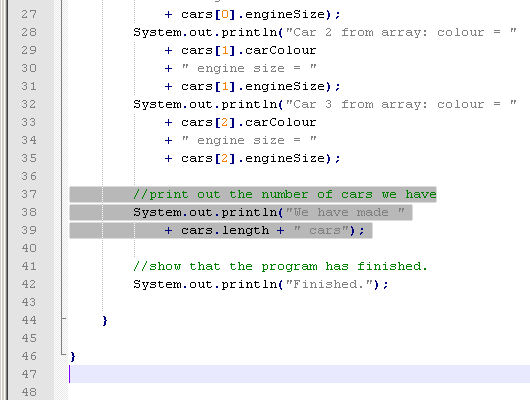
Introducing for-loops:
A for-loop is a block of code that will loop many times until a specified condition is satisfied. There are two different ways to syntactically write a for-loop. This practical is going to introduce and use the original format however we will see examples of the newer format later in this course.
There are some obvious advantages to being able to loop over a section of code many times. We can write a small section of code to do a repetitive task and simply loop over it many times rather than writing code to do each task repetition.
However, there are also some disadvantages, a serious one is that if the coder does not specify the conditions required to exit the for-loop correctly the code can get stuck in an infinite loop unable to progress!
for-loop Syntax:
There are essentially three sections to specifying the for-loop.
- Initialisation section: where a counter can be created. This is executed once as the for-loop begins.
- Termination or condition section: this is where an exit condition is set. When this section tests to false the loop is terminated. This section is tested before every loop is performed, even the first loop.
- Increment section: this is where the progression through the loop can be expressed. This section v is executed each time a loop is completed.
for(
Initialisation;
Termination;
Increment){
....code to loop over goes here....
}
.
Figure 17 shows two legal forms of for-loop. The first is a standard form and will result in any code
between the braces being iterated over 10 times. This is because we initialise a variable i
on entry setting it to equal 0. We then increment the variable i
after each iteration
using the i++
syntax. Finally, to exit the loop we have the conditional check,
i<10
so when i
increments to equal 10 this condition would become false and
the loop would exit.
The second loop format shown in Figure 17 is also valid. This is because all of the sections in the for loop syntax are optional. The syntax used in the second example in Figure 16 is very dangerous because there is no exit check and the for-loop would continue indefinitely.
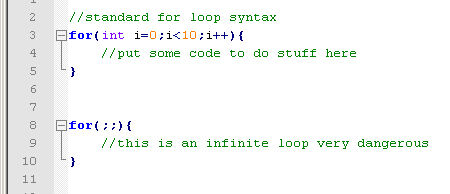
Combining for-loops and arrays:
Now it becomes interesting because what we can do is combine a for-loop with an array and what we have is a method to manage large quantities of data in one place and iterate over it with very little effort.
To demonstrate this lets alter our CarFactory.java file to use a for-loop to print
out the contents of the cars
array to the screen.
First delete the lines of code highlighted in Figure 18.
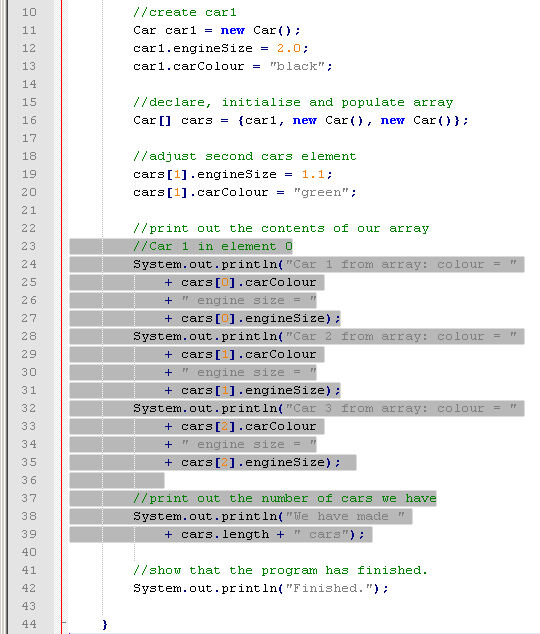
Now insert the lines as shown in Figure 19 to replace the ones we have just deleted.
Save, compile and run your program. The output hasn't changed, what we see in Figure 20 is the same as Figure 10 but we have reduced the amount of code required to achieve this output. This is very small example, but imagine if you had thousands or millions of elements in an array, the amount of code to cycle over them remains the same!
So how have we combined the array and the for-loop. We have initialised a counter i
in the for-loop at a value of 0. Which is, as we know, the base that the index of all arrays start.
The counter i
is incremented on each iteration, and when it is no longer less than
the length of the array (this line equates to false ;i<cars.length;
) the for-loop
exits.
Inside the for-loop we use the counter i
to supply the index for the element we want
to access from the array, cars[i]
. The index of the array has to be an integer, but
because we specified i
as the primitive data type int
we can use it
as the array index. A simple but very powerful pattern of code that you will use and see very often.
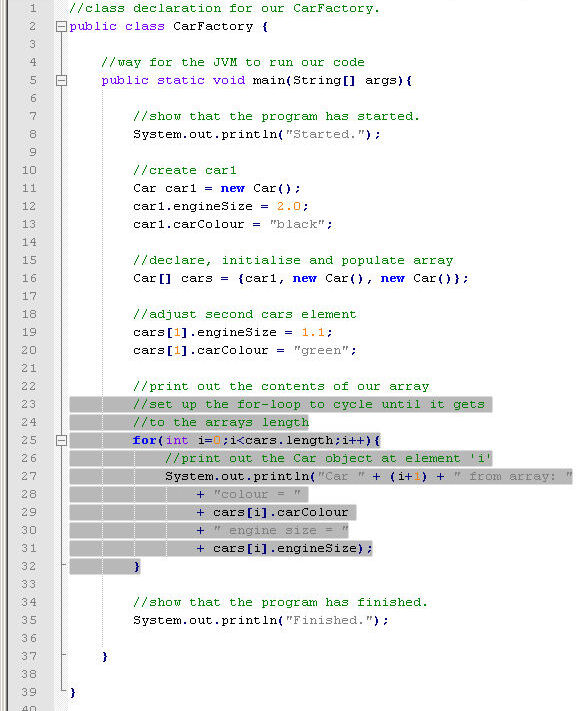
Introducing Multidimensional Arrays:
So far all of the examples we have looked at have been single dimensional (only have one index). It
possible and quite common to have multi-dimensional arrays. These are arrays of arrays. Figure 17
shows the structure of a two dimensional String
array called array2D
.
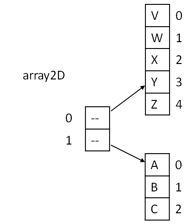
To declare, construct and initialise this array using all three methods you would use the following code
- Declare, construct and initialise separately
- String[][] array2D;
- array2D = new String[2][];
- array2D[0] = new String[5];
- array2D[1] = new String[3];
- array2D[0][0] = "V";
- array2D[0][1] = "W";
- array2D[0][2] = "X";
- array2D[0][3] = "Y";
- array2D[0][4] = "Z";
- array2D[1][0] = "A";
- array2D[1][1] = "B";
- array2D[1][2] = "C";
-
Declare and construct on one line
- String[][] array2D = new String[2][];
- array2D[0] = new String[5];
- array2D[1] = new String[3];
- array2D[0][0] = "V";
- array2D[0][1] = "W";
- array2D[0][2] = "X";
- array2D[0][3] = "Y";
- array2D[0][4] = "Z";
- array2D[1][0] = "A";
- array2D[1][1] = "B";
- array2D[1][2] = "C";
-
Declare, construct and initialise on one line
- String[][] array2D = {{"V","W","X","Y","Z"},{"A","B","C"}};
This all looks very daunting but if you remember that a multidimensional array is just an array of arrays it makes the concepts much easier to use. We don't have to stay at 2 dimensions we can increase the number of dimensions. It is a challenge when we begin to work with anything past 3 and there is a consideration for making your code readable to other developers that may need to follow what you have done.
We will revisit multi-dimensional arrays several times as we move through the course and you will become more confident and comfortable with them as we go and actually use them in real social science modelling applications.
Summary:
- An array can hold many objects or primitives of the same type in elements under one 'variable' name.
- Accessing the elements of an array is done using the index in square brackets
[]
. - The index of an array is an integer value.
- The index of an array always starts at 0.
- The size of an array is the number of elements it contains, this can be accessed using the
arrayName.length
attribute. - There are three main ways to declare an array.
- The type used in the declaration and construction must be the same and is the data type to be held in the array.
- Once an array has been constructed its length or size (number of elements) cannot be changed.
- Multi-dimension arrays are arrays of arrays.
- Multi-dimension arrays can only hold one data type (whether primitive or object).
- When an array is constructed the length must be specified.
- When constructing a multi-dimension array only the length of the first index is required.
- When declaring and constructing a multi-dimension array the number of dimension must be reflected by the number of pairs of square brackets
- The syntax for a for-loop has three main parts, initialisation, termination and increment.
- It is very dangerous to use a for loop without a satisfactory termination clause, you may find your code in an infinite loop.
- We can use the length property from an array as the termination clause in a for loop.
- So long as the for-loop counter is declared as an integer (
int
) variable it can be used as the index of an array.