Classes, Objects and Static
[Practical 2 of 11 - Part 2]
Static Revisited:
OK so now let’s see what all the fuss is about this key word static
.
First of all we are going to set up our Car
class to have some default values.
All the cars that are made in our factory are sprayed white unless otherwise stated and have
a 1.0 litre engine for super fuel efficiency.
Type in the two new lines highlighted in the screen shot shown in Figure 12 and alter the two lines that hold our engine size and car colour to set them to be the default, also highlighted.
So what are we doing here?
- Firstly we are setting two variables that hold a default colour
and engine size
(
defaultCarColour
anddefaultEngineSize
). When we create the variables we are giving them a value on the same line, just like we did when we created theCar
objects. This time the values are typed straight in and are not the name of a class. These are called literals and often referred to as hard coded values. - Secondly, when we create our
engineSize
andcarColour
variables we assign them whatever is in our default variables.
Lets save, compile and run our program again. No change… but we set the colour and engine size to the default values! So what’s going on?
When we first set up our car object by creating it (new Car()
) the default values
are assigned for the car colour and engine size, but later on in the CarFactory code we are
setting new engine sizes and colour for our cars which replace the current set values of
1.0 and ‘white’.
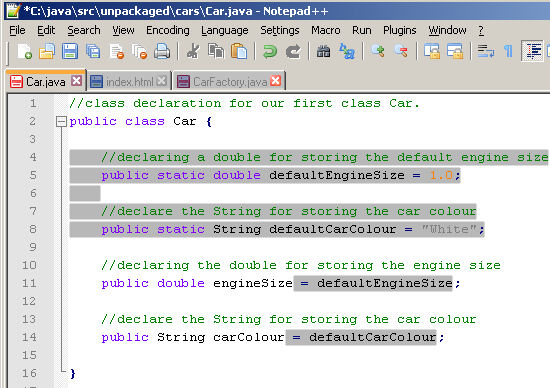
Testing the Defaults
OK so to prove that the defaults are there let’s create another Car
, car3
, but this time we won’t set any colour or engine size.
In your CarFactory.java
file add the lines highlighted in the screen shot in Figure 13.
Now compile and run the code.
You should have a third car added to the output which has the default colour and the default engine size.
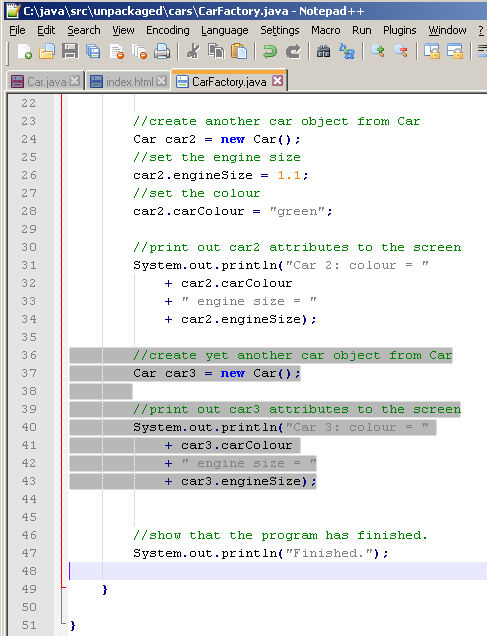
So let’s check the default colour and engine size for all of our cars.
Add the lines highlighted in the screen shot in Figure 14 to your CarFactory.java
file,
compile and run your program.
OK you should see all of the same outputs as before with the default colour and engine size for each of the cars listed below the original output, these should all be 'white' and 1.0 litre as shown in Figure 15.
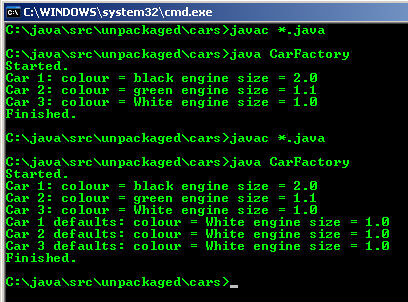
OK so what happens if the default car colour is changed to 'red' after the first Car is created?
What do you think will happen?
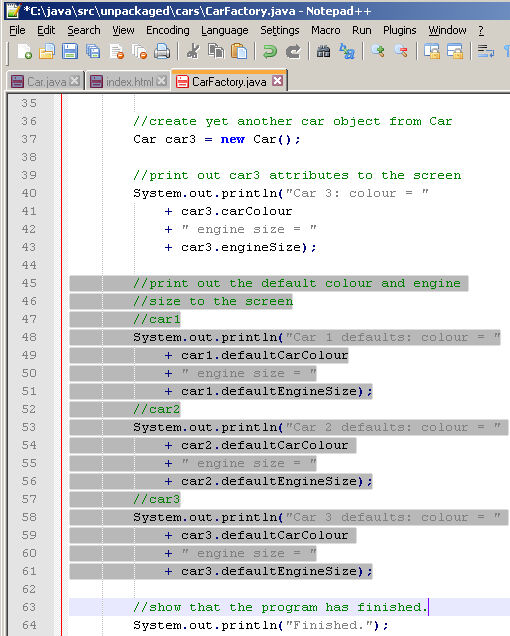
Lets see. Make the change highlighted in the screen shot in Figure 16, save, compile and run the code.
You should get the output shown in Figure 17.
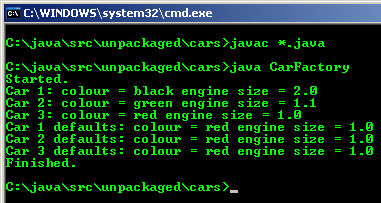
Even though car1
was instantiated before we changed the default attributes for
our Car
class it's default values have changed.
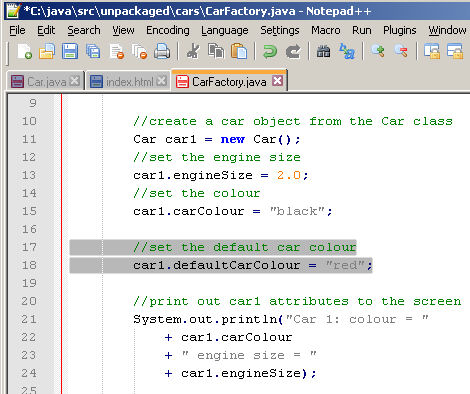
This is because there is only one class and the keyword static
we used when we declared our default variables means that they are class level
which means they belong to the class, not the object. All of the objects instantiated from
the Car
class can use these variables because they are based on that class.
When we adjust these variables they change for all of the objects based on the class!
The variables declared without using the static
keyword can change independently
as we have seen. These variables belong to the object and each object has its
own 'version' of these variables. These are referred to as instance level
attributes or variables.
In fact it is bad practice to use an object to change a static variable, we should use the class reference directly to make it explicit what we are doing, so change the last line we added to CarFactory as shown highlighted in Figure 18.
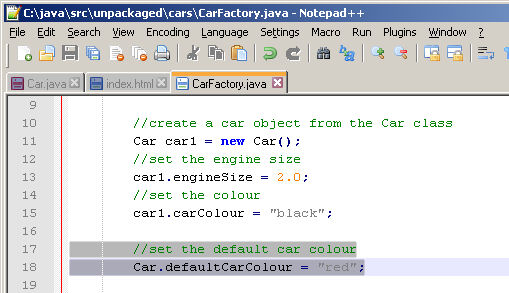
When we save, compile and run our program we should get no change to the results but it is now clear in the code that the variable does not belong to an object but to the class.
Note: if you try to access an instance level variable using a class reference your code will not compile. You will get an error like the one in Figure 19.
This is a result of trying to use the instance level variable carColour
from a reference to the class Car
and not an object based on the class such as
car1
, car2
or car3
.
So you can use a class level variable from an instance because the class always
exists (although it is bad practice, we should always reference the class
directly).
You cannot use an instance level variable from a
class because an instance may not exist and even if it does the class is not aware of it.
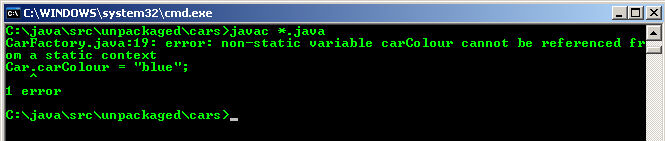
Summary:
We hope this practical has been useful. It has shown that:
- All objects are based on a class.
- Many objects can be created from one class.
- Objects are independent of each other when you change values within them. This is part of what we refer to in the programming world as encapsulation.
- The static keyword means that a variable is tied to the class and not an instance of that class (an object).
- Variables declared in a class that are not static are called instance variables (or attributes).
- Variables declared in a class that are static are called class variables (or attributes).