Data storage
[Framework practical 1 of 7]
Essentially, over these practicals we're going to build up an application that:
- Has a windows interface.
- Opens data files and reads in data.
- Displays the data as an image.
- Can write data out.
As you can see, this will then give you the framework of code to slot processing into.
We'll also have a separate practical on documenting code which will help us comment and document the framework.
[NB: although the application will ultimately look like that on the right, for the first few weeks it'll just print stuff to the command line -- we *will* get to windows, but we need some basics under our belts first.]
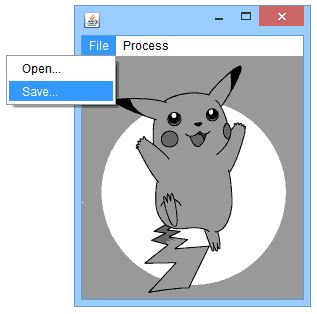
It is important you keep up with the practicals -- they build up to the final projects, so you won't get far without doing them all. Please, therefore, feel free to ask as many questions as you need. We won't write them for you, but they are meant to be practice, not a horrific experience.
In this practical we're going to build a main class, and give it an object variable in which it can store a 2D array of data. This is going to be our data store for later versions of the software. Here's the algorithms for the two classes we'll build:
Analyst class:
|
Storage class:
|
Start by copying your HelloWorld file to a new directory: GEOG5XXXM\src\unpackaged\framework1. Again, GEOG5XXXM should be replaced with your course code if you have one, or just something like java if you don't.
Each practical we'll take a fresh copy of the code to work on, so you can always backtrack to something that works and see how the projects build up. Once you've got the code copied, change the class name to Analyst, and the filename to match. Compile the code and check it works.
Why have we done something so simple? As we said in the last lecture, the best way to code is to build up a few lines at a time, and compile regularly. We've built and tested something so simple so we can now have confidence to build on it.
Now build a second class, "Storage" in a second file, "Storage.java". It doesn't need a main block, just an empty class block.
Again, compile the files - this time using "javac *.java" to compile both files.
Now make an object of type "Storage" in the main block of the Analyst class. Call it "store" (lower case), so we know what it is (we could call it anything - "harry" if we wanted - it just wouldn't be very helpful).
Again, compile the files.
We're now going to make the variable that is going to store our data.
Ultimately this is going to be an array, however, for the moment, let's make it a simple primitive variable. Make a "double" type variable called "data" in the "Storage" class. Make it equal to zero.
In the Analyst class, after the point where the Storage object called "store" is made, use the dot operator to
change the value of the "data" variable inside the "store" object to 10.0. Compile the code. If you're unsure how to do this, see the Point.x
example given in the lecture materials.
Now, in the Analyst class, after you've set the value of the double, add a System.out.println
line that gets the value of the "data" variable back out of the Storage object, and tests that it was set to 10.0. Compile and run the code to test it works.
Note that it is always worth thinking about how you would test any code you write to make sure it is working ok.
We'll now change the data variable in the "Storage" class so it is an array.
Adjust the code in the Storage class so the data variable is an array with 3x4 spaces of type "double".
In the Analyst class, adjust the code that uses the Storage's "data" object so it works with the new array format to set and get the value in the array at position "[0][0]". Compile and run the code to test it.
Now, from the Analyst class, fill the rest of the "data" array inside the Storage object with data.
In Analyst class, after the point where you've made and filled the data array, add the following code:
System.out.println("Number at [0][0] = " + store.data[0][0]);
System.out.println("Number at [1][1] = " + store.data[1][1]);
System.out.println("Number at [3][2] = " + store.data[3][2]);
If any of these lines don't work, why do you think this is? Fix it.
Once you've finished that lot you're done for this practical. Next practical we'll look at how we cope with manipulating thousands of numbers in our data array. In the meantime, if you are feeling like more practice, We've listed some coding problems for each week that will help you cut your teeth - you can find them on the "Practice Pieces" page linked on the main course page (right note).
Remember, learning programming is all about practice -- you can't just cruise through the lectures and practicals. Set aside some time each week for experimenting. Feel free to drop Andy a line at any time if you'd like to talk through problems.